Introduction to Node.js + MongoDB
"for easily building fast, scalable network applications" Node.js is sponsored by Joyent
Install Node.js
On OS X/Ubuntu check out nvm - Node Version Manager
Node.js Documentation
Node.js is *NOT* just for server-side apps. The Adobe Anyware Components team uses Node.js + Grunt as their build process. Node will uglify, minify and test their js code, but there's no "server" in those commands.
Node.js dependency management with npm
The real power for Node, like other contemporary languages, comes from dependency management. This enables rapid prototyping and experimentation. Node.js dependency management is in the form of 'npm' - Node Packaged Modules
Like I said with Chef in the previous meeting - it's all been done before!
But some libraries are better than others. Check for key stats like
- Number of downloads
- Number of stars
- How often it is dependened upon
Soooo - npm makes you work for it a little bit.
How to install a module?
npm install
local or-g
global install a single module- A local package is in the
node_modules
folder in your project - A global install will put the binary from npm in your $PATH
- Your project's
package.json
file --save-dev
or--save
will update your package.json file and thennpm install
- Sometimes you'll have to delete your
node_modules
folder when you're refactoring code
YOUR node_modules
ARE YOUR node_modules
, NOT MINE!
Several modules will compile themselves for your machine. So - checking the node_modules
folder in to Git is a bad move. Windows modules will not work on OS X. OS X modules will not work in Linux.
That said, if your build server (Jenkins) is running the same platform as you are deploying your application too, feel free to scp or rsync the node_modules
directory when deploying your app.
Long story short. You'll have a line for node_modules
in your projects .gitignore
file.
Benefits of Node.js
- Generally all code in the app, client and server-side will be JavaScript. Familiarity with JS enables developers to look at the whole stack.
- Vibrant community - npm libraries are *generally* kept up to date. Or someone will fork it and pick up the torch.
- Like Chef community, modules are mostly open source and on GitHub! Need a feature, ask for it or create it and send the Pull Request.
- Downside: JS developers (as a stereotype) *love* to reinvent the wheel...
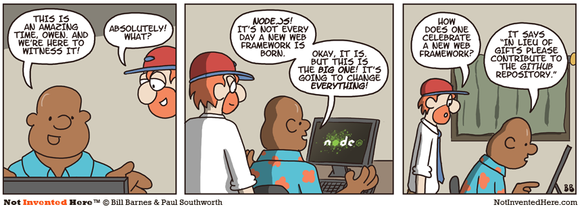
Developing with Node.js
What's the life cycle for doing Node.js server-side app?
- Run your app with with node.
node app.js
- Until you make your first error...
- Bring on
nodemon
andforever
. Which one is better? The one that works for you. - And in Production environments? You'll want Passenger from Phusion. Which will hook in to nginx or Apache and ensure your node process is running and served out by a proper webserver.
Further Node.js learning
Cuddle up with a nice book.
Benefits of MongoDB
- Free! *cough* Oracle *cough*
- Rapid application protoyping
- Stores documents like JSON, so plays very well with JS apps
- Scales vertically and horizontally
- Replication
- Sharding
Setting up MongoDB
You love your terminal right? Because MongoDB doesn't do much with GUI interfaces.
Querying MongoDB
Your data is in MongoDB. You'll want to get it out.
With Node.js I use the mongodb
module. A query (find) looks like this
string_collection.find()
string_collection.find({stringCode:'developers',localeCode:'en'})
Further MongoDB learning
Enroll (for free) at The MongoDB University.
Self-paced learning to use MongoDB in your preferred programming language (Node.js or Java), or learn to adminster a server.
Workout
We are going to complete the following actions
- Check out the example app
- Start up the Vagrant box.
vagrant up
and let Chef install Node.js and MongoDB for you. - SSH in to your Vagrant box.
vagrant ssh
- Install your Node modules and start the app
cd /apps/node
npm install
node app.js - Install
nodemon
as a global module on the VM. Runapp.js
usingnodemon
- Add your name to the 'developers' section of the
app/routes/index.js
file query the REST API for it. Developers string group - Send your file change back as a Pull Request where you forked it from!